Understanding Typescript symbols `&&`, `||`, `??`, `?.`
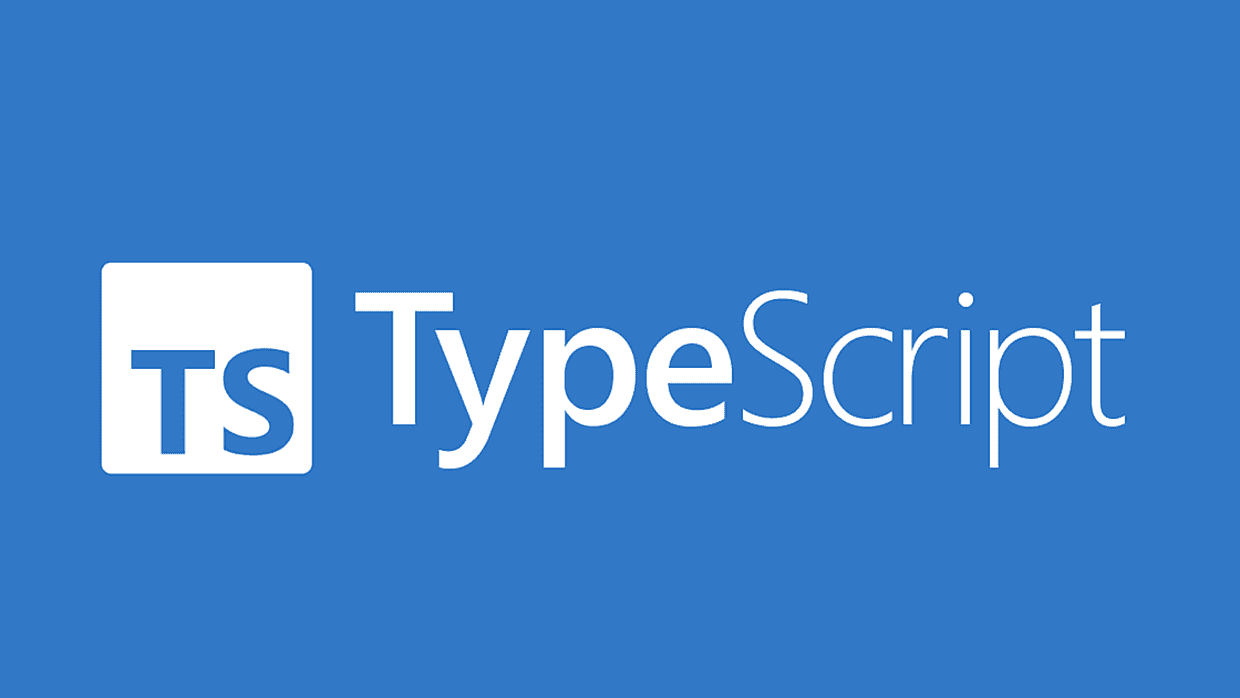
In TypeScript, the &&
, ||
, ??
, and ?.
operators are used to handle logical operations, nullish coalescing, and optional chaining. Here’s an explanation of each:
1. Logical AND (&&)
The `&& operator is known as the logical AND. It evaluates expressions from left to right and returns the first falsy value it encounters, or the last value if all are truthy.
const a = 1 && 0; // 0
const b = null && 1; // null
const c = "hello" && "world"; // "world"
In these examples:
- a evaluates to
0
because1
is converted to truthy value while0
is falsy value. - b evaluates to
null
becausenull
is a falsy value. - c evaluates to
world
because both 'hello' and 'world' are truthy, so it returns the last value.
Practical usage:
- Use the
&&
operator to conditionally execute a piece of code only if a certain condition is true.
function Home() {
const [isLoading, setIsLoading] = useState(false);
return isLoading && <p>Loading...</p>;
}
2. Logical OR (||)
The ||
operator is known as the logical OR. It evaluates expressions from left to right and returns the first truthy value it encounters, or the last value if all are falsy.
const a = undefined || null; // null
const b = null || 1; // 1
const c = "hello" || "world"; // "hello"
In these examples:
- a evaluates to
null
because bothundefined
andnull
are falsy, so it returns the last value. - b evaluates to
1
becausenull
is a falsy value. - c evaluates to
hello
becausehello
is the first truthy value in the expression.
Practical usage:
- Use the
&&
operator to provide a default value for falsy values.
function greet(name) {
const userName = name || "Guest";
console.log(`Hello, ${userName}`);
}
greet('Alice'); // Output: Hello, Alice
greet(''); // Output: Hello, Guest
3. Nullish Coalescing (??)
The ??
operator is known as the nullish coalescing operator, introduced in ECMAScript 2020. Similar to ||
operator, it returns the value of the right-hand side operand when its left-hand side operand is either null
or undefined
, but it does not apply for any other falsy values.
const a = undefined ?? "default"; // "default"
const b = 0 ?? 1; // 0
In these examples:
- a evaluates to
default
because the left-hand side operand isundefined
and it returns its right-hand side's value. - b evaluates to
0
because0
is a falsy value.
4. Optional Chaining (?.)
The ?.
operator, also introduced in ECMAScript 2020, is the optional chaining operator. It allows you to safely access deeply nested properties of an object without causing runtime errors.
let user = null;
const address1 = user?.address; // undefined
user = {
address: "123 Main St",
};
const address2 = user?.address; // "123 Main St"
In these examples:
- address1 evaluates to
undefined
because user isnull
and accessing theaddress
property returns immediatelyundefined
without causing runtim error. - address2 returns the value of the
address
property.
Practical usage:
- Combine
?.
and??
operators to safely access nested properties of the object and provide a default value if none of the values are found.
let user = null;
const address = user?.address ?? "Unknown Address";