How to setup a Typescript project
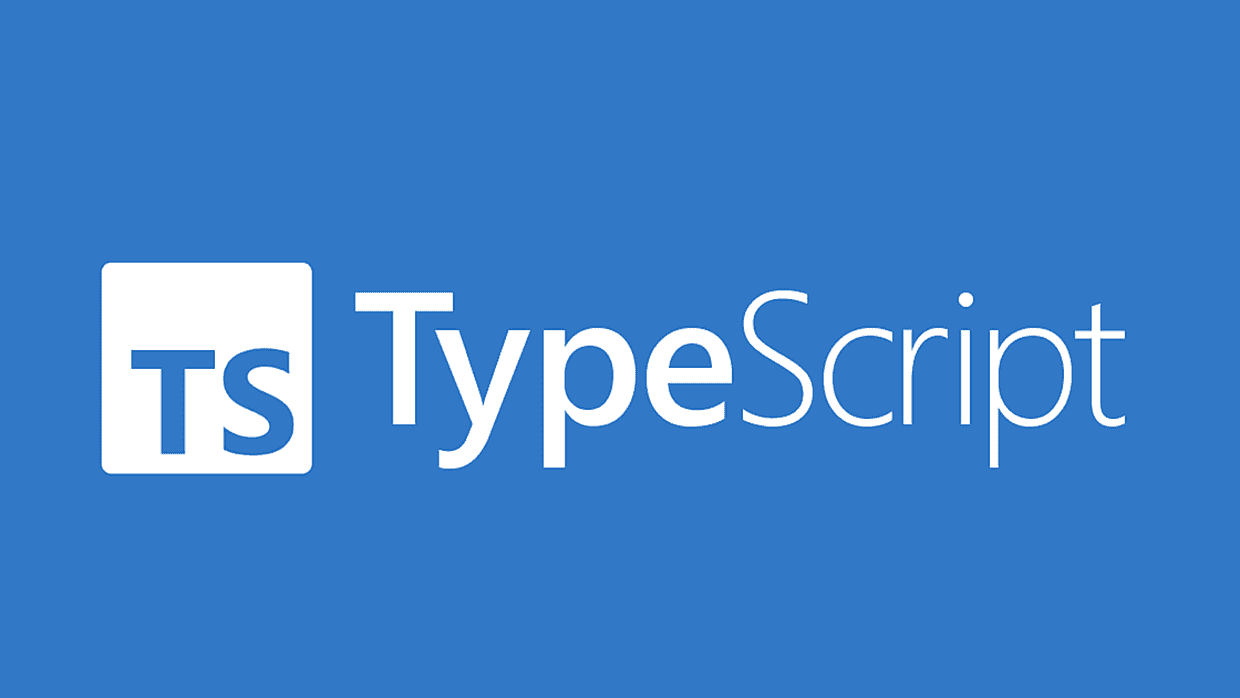
Introduction
TypeScript enhances JavaScript by introducing static typing, which makes it easier to detect problems and write dependable code. TypeScript has become a popular solution for developing scalable and stable apps because of its robust developer tools and support for contemporary JavaScript features. This post will show you how to quickly set up a Typescript project from the ground up.
Prerequisites
- Node.js
- Npm
Step-by-step guide
- Create a new directory for your project and navigate into it
mkdir my-typescript-project
cd my-typescript-project
- Create a
package.json
file with default settings and add Typescript to your project
npm init -y
npm install --save-dev typescript
- Generate a
tsconfig.json file
, which tells TypeScript how to compile your code:
npx tsc --init
Then modify it as needed; here’s a common configuration:
{
"compilerOptions": {
"target": "es6",
"module": "commonjs",
"strict": true,
"esModuleInterop": true,
"skipLibCheck": true,
"outDir": "./dist",
"rootDir": "./src"
},
"exclude": ["node_modules"]
}
- Create a
src
and adist
folders in your project root
mkdir src dist
Inside the src
, create a sample file index.ts
const message: string = "Hello, TypeScript!";
console.log(message);
- To automate the running code, install the following packages
npm install --save-dev nodemon ts-node
- Update the
package.json
with the following scripts
"scripts": {
"build": "tsc",
"start": "node dist/index.js",
"dev": "nodemon --watch src --exec ts-node src/index.ts"
},
You can run your project with live-reloading
npm run dev
Conclusion
With this setup, you're ready to use TypeScript's type safety and powerful development features to create robust and scalable apps.